3D online games and apps can improve a website by making it more engaging, interactive, and interactive. By incorporating 3D elements, a website can provide users with a more immersive and realistic experience, which can help to increase engagement and retention. For example, a website that sells furniture could use 3D web apps to create virtual room planners and configurators, allowing customers to visualize how different furniture pieces would look in their own homes.
In terms of interactivity, 3D web apps can create interactive product demonstrations, virtual tours, and other tools that can help to increase user engagement and conversions. For example, a website that sells cars could use 3D web apps to create interactive virtual test drives, allowing customers to see and experience the car in a more realistic way.
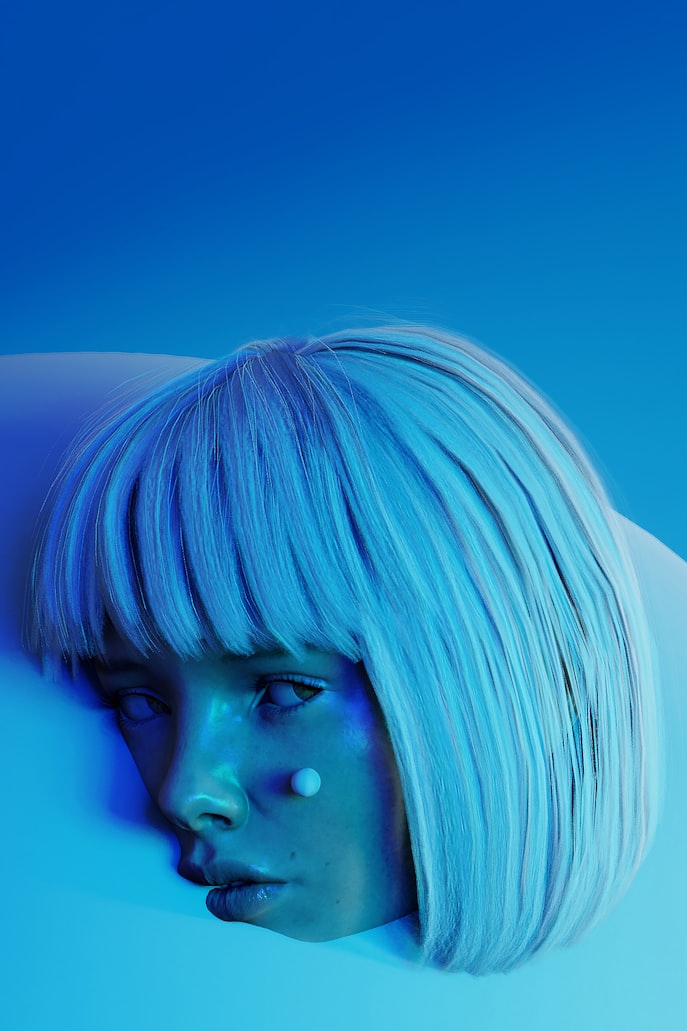
3D web apps can also be used to create interactive data visualizations, which can make it easier for users to understand and interact with complex data. For example, a website that provides financial analysis could use 3D web apps to create interactive charts and graphs, making it easier for users to understand market trends and make better investment decisions.
In terms of customer service, 3D web apps can be used to create virtual customer service tools, which can improve customer service and support. For example, a website that sells appliances could use 3D web apps to create virtual troubleshooters, which can help customers identify and solve problems with their appliances.
Finally, 3D web apps can be used to create interactive product design tools, which can improve product development processes. For example, a website that sells clothing could use 3D web apps to create virtual fitting rooms, allowing customers to try on clothes and see how they look before making a purchase.
In summary, 3D online games and apps can improve a website by making it more engaging, interactive and interactive, which can help to increase user engagement, conversions, and customer service. 3D web apps can be used to create virtual product demonstrations, product configurators, interactive data visualizations, virtual customer service tools, and interactive product design tools, all of which can provide a more engaging, interactive and interactive experience for the users and help to increase user engagement, conversions and customer service.